Enhancing API Security: Safeguarding Sensitive Data Exposed by APIs
Tl;dr
- API security is vital for protecting sensitive data exposed by APIs
- Following a comprehensive API security framework is crucial
- Key aspects include API discovery, analysis, detection and prevention, authentication, access control, and encryption
- API encryption closes a critical gap in protection, keeping data encrypted even in cases of unauthorized access
- Prioritizing API security and implementing robust encryption measures are essential for safeguarding data integrity and confidentiality
Application Programming Interface (API) security is all the rage, and as with any technical security discipline, it has lots of hype, but rarely any “magic” that will render your APIs secure and impenetrable. In this content, we’ll discuss various types of APIs, general API-related security practices, and discuss how you can protect sensitive data exposed by APIs.
API Security Framework and Common Capabilities
Most API security solutions in the market today are designed to protect the security and integrity of Application Programming Interfaces (APIs) and typically follow a general framework and have similar capabilities.

Discover: Identify and catalog all APIs within your environment to gain a comprehensive understanding of your API landscape.
Analyze: Assess the security posture of your APIs, evaluating vulnerabilities and potential risks.
Detect: Employ monitoring and detection techniques to identify suspicious activities, potential breaches, or anomalies within API traffic.
Prevent: React to attacks in real-time, in order to mitigate the impact of an attack.
Test: Conduct regular security assessments and penetration testing to evaluate the security of APIs.
It's important to note that different API security products may offer varying sets of features and functionalities to secure APIs, but these are some common aspects you'll find in many API security solutions.
- API Discovery and Identification: API security products often include features to aid in the discovery and identification of APIs within an organization's ecosystem. These tools can scan networks, applications, and documentation to identify existing APIs and create an inventory of available endpoints. They may employ techniques such as static analysis, network sniffing, or integration with API management platforms to gather information about APIs deployed within an organization.
- API Inventory Management: API security products provide capabilities for managing and maintaining an inventory of the organization's APIs. They help track the details and metadata of each API, including versioning, endpoints, authentication mechanisms, access controls, and dependencies. This inventory management functionality enables organizations to have a comprehensive view of their APIs, facilitating better governance, documentation, and security management.
- Vulnerability Detection: API security products often incorporate features for detecting vulnerabilities in existing APIs. These tools can perform security assessments and vulnerability scans to identify potential weaknesses or misconfigurations in the API implementation. They may check for common vulnerabilities such as injection attacks, broken authentication and session management, insecure direct object references, and more. By identifying these vulnerabilities, organizations can take proactive measures to mitigate risks and protect their APIs.
- Authentication and Authorization: API security products facilitate authentication and authorization mechanisms to ensure that only authorized users or systems can access the API. This can involve methods such as API keys, tokens, OAuth, or other authentication protocols.
- Access Control: API security products enforce access control policies to determine what actions or resources an authenticated user or system can access. They define and enforce permissions and restrictions to prevent unauthorized access to sensitive data or functionalities.
- Rate Limiting: These products implement rate limiting mechanisms to prevent abuse or overuse of APIs. Rate limits help control the number of requests a client can make within a specific timeframe, protecting the API server from excessive traffic or potential attacks.
- Data Validation and Filtering: API security products validate and filter incoming API requests to ensure that the data being transmitted is in the expected format and adheres to defined rules. This helps prevent common security vulnerabilities such as injection attacks, cross-site scripting (XSS), or SQL injection.
- Threat Detection and Prevention: API security products often employ advanced threat detection techniques to identify and block suspicious activities or potential security breaches. They can detect patterns of behavior indicative of attacks, such as brute force attempts, abnormal usage patterns, or malicious payloads.
- Security Testing and Penetration Testing: API security products may offer capabilities for security testing and penetration testing of APIs. These features simulate real-world attacks against the API to identify potential security weaknesses. Security testing involves assessing the API's resilience to various attack vectors, whereas penetration testing involves attempting to exploit vulnerabilities to gain unauthorized access or extract sensitive data. By conducting such tests, organizations can proactively identify and address security gaps before malicious actors exploit them.
Understanding the roles of APIs
The primary purpose of APIs is to enable communication and interaction between different software systems, allowing them to exchange data and functionality. APIs serve as intermediaries that define the rules and protocols for how different software components can interact with each other.
APIs expose specific features, services, or data of a software system to other applications or developers. They provide a standardized interface that hides the underlying complexity and implementation details of the system. This allows developers to utilize the functionality of a software system without needing to understand its internal workings.
APIs can share or expose various types of resources and functionalities, depending on the system they represent. Here are some common examples:
- Data APIs: These APIs expose access to data resources, allowing other applications to retrieve, create, update, or delete data. Examples include APIs provided by social media platforms, e-commerce websites, or banking systems to retrieve user information, product data, transaction history, etc.
- Service APIs: These APIs expose specific functionalities or services provided by a software system. For example, a payment gateway API enables other applications to process online payments, while a geolocation API provides services to determine the location of a user or device.
- Integration APIs: These APIs facilitate the integration of different software systems by exposing interfaces to exchange data or trigger actions between them. They enable systems to communicate and share information in a coordinated and automated manner. For instance, APIs used for integrating customer relationship management (CRM) systems with email marketing platforms or inventory management systems.
- Framework/API Libraries: These APIs provide a set of pre-built functions, classes, or modules that developers can use to accelerate their application development. Framework APIs, such as those provided by web frameworks like Django or Ruby on Rails, offer ready-to-use components for building web applications, handling HTTP requests, database interactions, and more.
- Third-Party APIs: Many companies and services provide APIs that allow developers to integrate their applications with external functionalities or platforms. Examples include social media APIs (e.g., Facebook, Twitter) that enable developers to incorporate social sharing or authentication into their applications, or weather APIs that provide weather data for specific locations.
For the purposes of this content, we’ll focus primarily on protecting data within Data APIs, but the concepts we’ll discuss can apply to most APIs.
API Data Encryption with Ubiq
Since one of the primary purposes of APIs is to exchange data and the primary purpose of most cybersecurity products is to protect data, we believe it’s critically important to ensure your APIs are encrypted.
When encrypting data with Ubiq, we don’t care what data you’re encrypting or where that data lives. In at-rest encryption scenarios, the data is usually in an unstructured bucket/filesystem of some kind or in a structured database. When securing APIs, however, the data being encrypted is not “at rest,” but instead it is the data that is exposed by the API in its payload. As with all kinds of encryption, this requires that both the service (serving the API) and the consumer (the client calling the API) are able to encrypt and decrypt so they can effectively share that data.
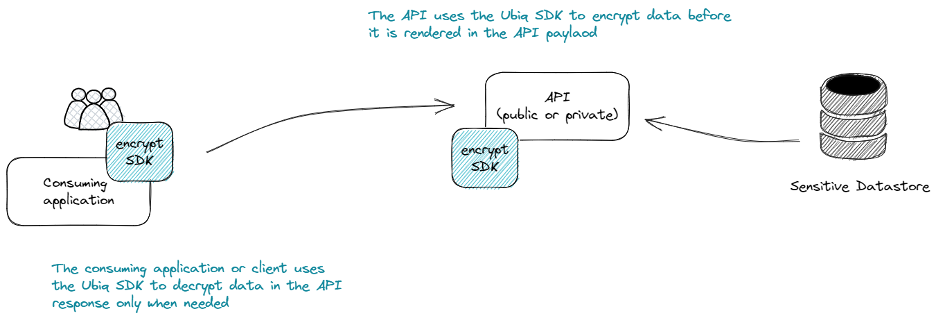
By implementing encryption at the API and decryption at the consuming application / client, data exposed by the API is protected against unintended exposure, unauthorized access, or breaches. Even if your perimeter security is compromised and a protected API is accessed, the attacker or accidental exposure will only see ciphertext.
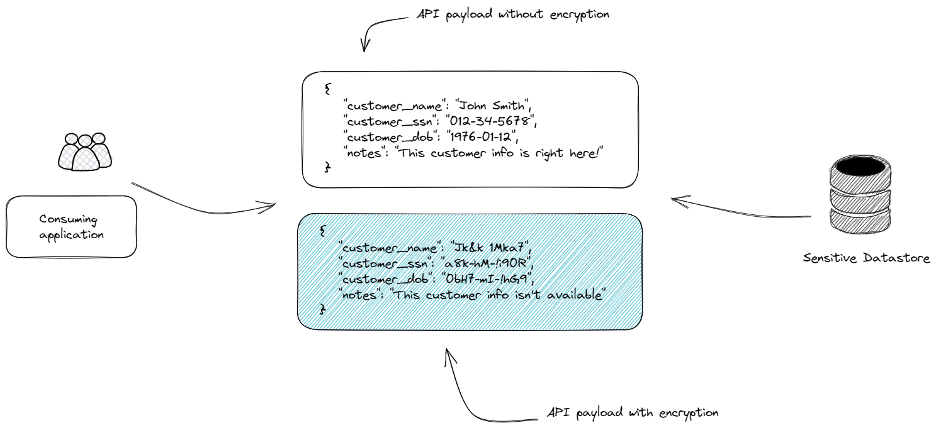
Granular Control Over Data
Once the data in your API payloads are encrypted, you can control what data is shared both at the API authorization layer, but also at the decryption/key layer. Even if the API is accessible to a client or consumer, they need access to decrypt it in order to be exposed to sensitive data. Without decryption access, they can get to the API but they can’t see the data. Access for decryption can be controlled granularly through Ubiq at the attribute level or the consumer level.
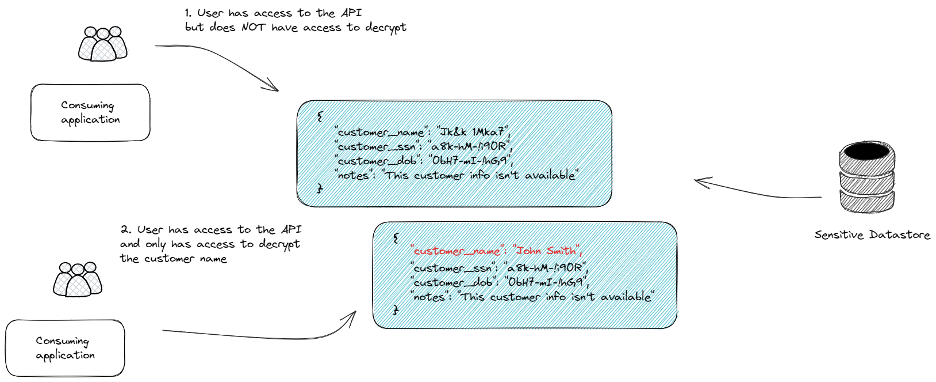
Added Value of API Data Encryption: Data Masking and Reusability
With the primary goal of data protection achieved by encrypting the payload of the API, using encrypted data also enables other efficiencies in your Software Development Lifecycle (SDLC) and DevOps processes:
- Data masking via structured data encryption. As discussed in our structured vs. unstructured and data protection types docs, structured data encryption provides a better alternative to data masking. If your API payload data is encrypted, you can leave some encrypted (not decryptable by clients) to provide in-place masking.
- Re-usable data in non-production environments. Without the keys to decrypt, data is safe, making it simpler to create test or sample data by using the ciphertext directly.
Case Study: Optus Data Breach and API Encryption
The Optus data breach serves as a cautionary example of the consequences that can arise from inadequately securing APIs. In this case, the breach occurred through an unprotected and publicly exposed API that did not require user authentication. Let's analyze the flaws that led to the breach and explore how implementing API encryption could have mitigated the risks and vulnerabilities.
Security Flaw #1: Public-Facing API
The first flaw was the use of a public-facing API that granted access to sensitive internal data. Traditional security best practices dictated that APIs should not be public-facing if they provide access to sensitive information or interact with core business operations, though the Zero Trust security movement is challenging this principle. By making the API publicly accessible, Optus exposed its customer data to potential threats.
Mitigation with API Encryption:
Implementing API encryption would have added an additional layer of security. By encrypting the data transmitted through the API, even if it were exposed, the data would remain protected. Encryption ensures that only authorized parties with the necessary decryption capabilities can access and decipher the sensitive information.
Security Flaw #2: Lack of Authentication and Access Control
The second flaw was the absence of user authentication and access control mechanisms in the API. Without requiring authentication, anyone who discovered the API could connect to it without submitting valid credentials, potentially exposing sensitive customer data.
Mitigation with API Encryption:
API encryption does not replace authentication and access control but can complement these measures. By implementing encryption alongside strong authentication mechanisms such as API keys, tokens, or OAuth, unauthorized access to the API could have been prevented. Encryption would have ensured that even if the authentication failed or was bypassed, the data exchanged between the API and the client would remain encrypted and unreadable to unauthorized individuals.
Security Flaw #3: Predictable Customer Identifiers
The third flaw was the use of incrementing customer identifiers that were easily predictable. By employing sequential identifiers, hackers were able to exploit the vulnerability more efficiently and extract data from multiple customer records without resorting to brute force techniques.
Mitigation with API Encryption:
While API encryption does not directly address the issue of predictable customer identifiers, it adds an extra layer of protection to mitigate the impact of such vulnerabilities. With API encryption, even if an attacker gains access to the customer database, the encrypted data remains unreadable without the appropriate decryption capabilities. This helps safeguard the sensitive information, limiting the potential damage caused by predictable customer identifiers.
By implementing API encryption in the Optus scenario, the breach could have been significantly mitigated. Encrypted data would have rendered any unauthorized access futile, protecting customer information even in the event of a successful breach. Additionally, encryption would have acted as an extra safeguard against the exposure of sensitive data through the publicly accessible API.
Enhancing the API Security Framework
It is essential for organizations to prioritize API security and consider implementing robust encryption measures to protect the confidentiality and integrity of data transmitted through their APIs. We believe that a comprehensive API security strategy must have data encryption as an elemental component to ensure data is adequately protected when APIs are inevitably exposed or improperly accessed.

Discover: Identify and catalog all APIs within your environment to gain a comprehensive understanding of your API landscape.
Analyze: Assess the security posture of your APIs, evaluating vulnerabilities and potential risks.
Encrypt: Implement encryption mechanisms to safeguard the confidentiality and integrity of data transmitted through APIs.
Detect: Employ monitoring and detection techniques to identify suspicious activities, potential breaches, or anomalies within API traffic.
Prevent: React to attacks in real-time, in order to mitigate the impact of an attack.
Test: Conduct regular security assessments and penetration testing to evaluate the security of APIs.
API security is a must-have in today's digital landscape. By implementing a robust API security framework and leveraging encryption to protect sensitive data, organizations can mitigate risks, prevent unauthorized access, and ensure the integrity and confidentiality of their API-driven interactions. Whether it's API discovery, vulnerability detection, authentication, access control, or encryption, each aspect plays a vital role in building a comprehensive API security strategy. By prioritizing API security and adopting strong encryption practices, organizations can confidently leverage the power of APIs while safeguarding their valuable data assets. Remember, protecting your APIs is not just a best practice; it's a crucial step towards maintaining trust, reputation, and ensuring the overall security of your digital ecosystem.
Updated 14 days ago